Webhooks and What They Are?
Unravel the mysteries of webhooks and discover their power in real-time app interactions. From basic definitions to hands-on Node.js examples, this post has you covered. Say goodbye to constant API polling and hello to event-driven design!
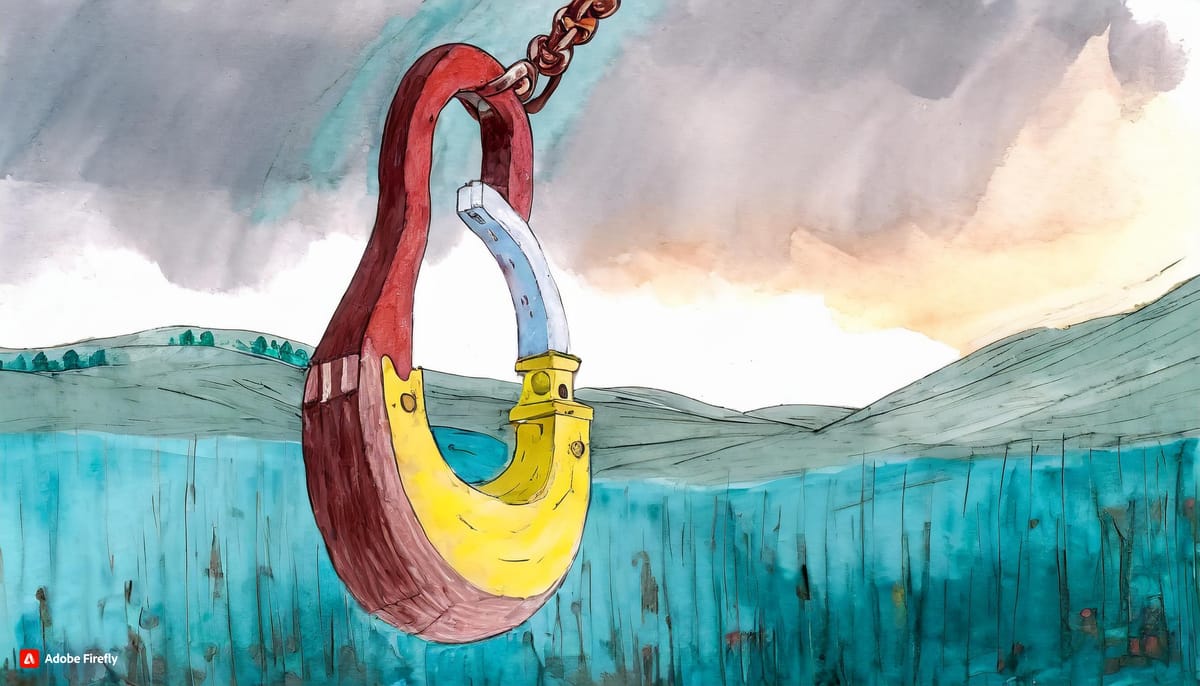
Hey everyone! If you've been dabbling in web development or software architecture, you've probably heard of the term "webhook" thrown around quite a bit. But what exactly is a webhook, and why should you care? In today's post, I'll demystify what webhooks are, why they're different from APIs, and why they're an essential tool in your developer arsenal. Stick around, because this is going to be one enlightening ride!
🤖 What's a Webhook?
Now let's get down to the nitty-gritty: What exactly is a webhook?
In its simplest form, a webhook is a way for one server to send real-time data to another server as soon as an event occurs. No need to ask, "Hey, got any updates for me?", webhooks bring the info right to your doorstep. This is basically why it's called a "hook", like when you're fishing!
Imagine you have a friend who always keeps you updated on the latest news. Instead of you constantly checking a News app (which is usually called "Polling"), your friend texts you the moment something big happens. In this scenario, a webhook acts like that friend, automatically sending you updates from one server to another as soon as there's new data or a specific event occurs.
Basic Mechanics
So, how does this doorbell, um, I mean webhook, work? Essentially, you set up a URL on your server where the webhook can "ring" to notify you. This URL is called an "endpoint," and it's where the data will be sent when the event you're interested in occurs.
So basically:
- Event Trigger: Something happens in the source system.
- Notification: The source system sends an HTTP POST request to your webhook URL.
- Action: Your server processes the data as needed.

Advanced mechanics
For those of you interested in the more advanced stuff, webhooks can also handle complex situations by incorporating various verification methods, custom headers, and even retry policies for failed deliveries.
- Signature Verification: Adds an extra layer of security by verifying the data's origin.
- Custom Headers & Payload: Allows you to include additional information in the header and/or the body of the HTTP request.
- Retry Policy: If your server fails to acknowledge the webhook's request, a retry mechanism ensures that the data eventually gets through.

🔄 API vs Webhook
Now that we know what a webhook is, you might be wondering, "How is it any different from an HTTP API?" Great question, and quite a legit one! Both are communication methods between servers, but they have their own distinct flavors. Let's break it down.
Definition of API
An API, or Application Programming Interface, acts like a menu in a restaurant. You look at the menu (the API documentation), decide what you want (the data), and ask the waiter (make an API request) to bring it to you. You have to initiate the action by asking; nothing comes to you unless you request it.
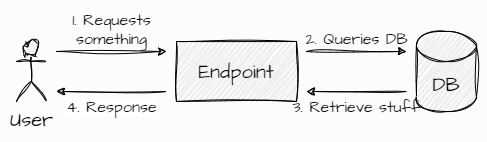
Key Differences
The main differences between a usual HTTP API and a Webhook, can be in three of their characteristics: How they are initiated, when they are processed, and what is their data flow direction.
API | Webhook | |
---|---|---|
Initiation | You ask for data | Data comes to you automatically |
Process time | On-demand, you pull the data when you want it | Real-Time, pushes the data instantly when and event occurs |
Data Flow | Uni-directional, you ask, you receive | Bi-directional, both systems may send and receive |
And that's how webhooks and APIs differ! In the next section, we'll dig into what you can actually do with webhooks, so don't go anywhere.
🛠️ Webhook Uses
You know what webhooks are and how they differ from APIs. Now, the million-dollar question: What do you actually do with webhooks? Well, let me tell you, they're useful in more ways than you can imagine!
Common Use-Cases
- Real-Time Notifications: Imagine getting an alert the instant someone signs up on your platform. No need to keep hitting refresh!
- Automated Workflows: Say you want to automatically add new email subscribers to your CRM. Webhooks make it seamless.
- Data Synchronization: Keep data consistent across multiple platforms in real-time.
- Event Logging: Track user behavior on your app and log it for analysis.
Sure, you can use webhooks for the usual stuff, like real-time notifications and automated workflows. But how about these creative uses?
- Dynamic Pricing Engine: A webhook updates e-commerce item prices in real-time, based on variables like demand or weather conditions.
- AI-Driven Customer Support: If a customer lingers on the FAQ page too long, a webhook triggers a chatbot session to assist.
- Content Curation: A webhook autopopulates a blog or news site with trending topics or related content.
- Gamification: Achievements or badges in an app unlock when users complete certain actions, thanks to a triggered webhook.
How is a Webhook made?
Before we jump into the code and build our first webhook, I want to make it clear, how a usual webhook is made. To be honest, it is not much different from a normal API endpoint.
First, one identifies the event that will serve as the webhook's trigger. It could be a new newsletter subscription, user click or a finalized purchase. This is the "trigger" in the webhook's life cycle.
Next, a designated URL on the server needs to be set up; this is the "endpoint." It serves as the receiving point for the webhook messages coming from the source system.
Once the endpoint is operational, the next step is to "register" it with the source system. This is where one enters the endpoint URL into the receiver system, thus establishing the webhook connection.
Before deploying, a test run is essential. The event that serves as the trigger is manually activated to ensure that the webhook behaves as intended and the server processes the incoming data correctly.
Once satisfied with the test results, it's time for the big move—deploying the webhook to the live environment. And just like that, the webhook is up and running!
So, webhooks not only offer staple functionalities but also present an array of creative possibilities. Coming up next, a dive into design patterns that elevate webhook implementation to the next level.
🏗️ Design Patterns
Now that you've got a solid understanding of what webhooks are and how you can get creative with them, let's talk about some design patterns that pair well with webhooks. Trust me, this is where the magic happens.
Event-Driven Architecture
First up is the Event-Driven Architecture. This makes a total sense, since webhooks are meant to react to events and this is your go-to if you're looking to build a super responsive and agile system. With this pattern, each component in your system is like an actor on a stage. When something notable happens—like a user clicking a "buy now" button—a message is broadcast to all components that might be interested. A webhook can act as the messenger, informing other parts of the system about this action.
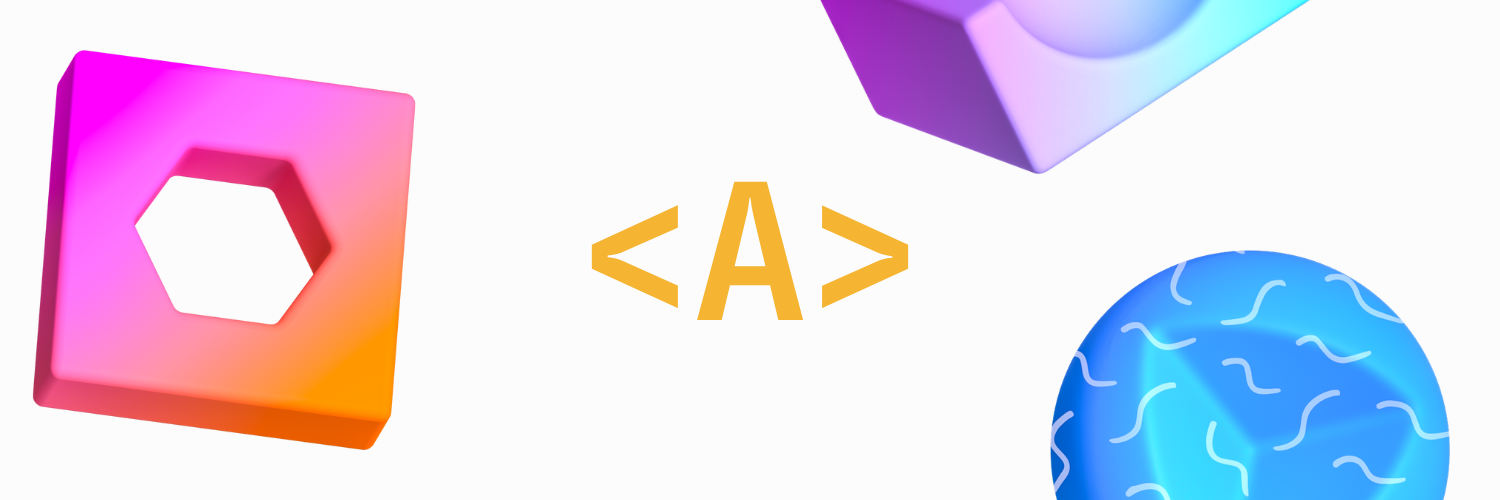
Observer Pattern
Next, let's talk about the Observer Pattern. This one's like having a bunch of fans following your every move on social media. Say you post a new photo; your fans are notified instantly. In tech terms, your 'subject' (like an inventory system) will notify all its 'observers' (like an email notification system) as soon as its state changes. Here, a webhook could be the perfect medium to send out these real-time updates.
So, whether you opt for an Event-Driven Architecture or lean more towards the Observer Pattern, integrating webhooks into these design patterns can seriously elevate your game. Next up, we'll roll up our sleeves and dive into some actual Node.js code to implement a webhook, so don't go away!
💻 Node.js Example
By now, you're probably itching to see how to actually implement a webhook in a Node.js app. Worry not, my friends, because you're about to dive into some juicy code snippets. Make sure you've got your favorite IDE open and Node.js installed! For that, I always recommend using NVM.
Project Setup
Let's, as always, start with the very basics.
mkdir webhook
cd webhook
npm init -y
We then use Express to quickly set up the routing etc.
npm install express
Now, we'll jump into the actual coding. We start by creating an index.js
file and add the following code to set up our basic Express app.
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('webhook app is up and running!');
});
app.listen(port, () => {
console.log(`app running at http://localhost:${port}`);
});
We then create a POST
route to handle incoming webhook data:
app.post('/webhook', (req, res) => {
console.log('received webhook data:', req.body);
res.status(200).send('OK');
});
To test it out, you can use tools like Postman to send a POST request to http://localhost:3000/webhook
and check your console to make sure the data is logged.
Our webhook setup is done. I mean, to be honest, that's it! As of now, we'll focus on more advanced topics of a webhook, it is validating the payload and event.
app.post('/webhook', (req, res) => {
const payload = req.body;
// Basic validation
if (!payload || !payload.event_type) {
res.status(400).send('bad Request: missing required fields');
return;
}
console.log(`received webhook for event: ${payload.event_type}`);
// Handle different events
switch (payload.event_type) {
case 'user_signup':
// Do something when a user signs up, like sending a welcome email
console.log('new user signed up:', payload.user);
break;
case 'order_placed':
// Handle a new order, maybe send it to the fulfillment center
console.log('new order placed:', payload.order);
break;
case 'payment_received':
// Update the order status when a payment is received
console.log('payment received:', payload.payment);
break;
default:
console.log('unknown event type, ignoring.');
}
res.status(200).send('Webhook received successfully');
});
See what I did there? Now, depending on the event_type
field in the payload
, we can perform different actions. In a real-world app, these could be things like sending out emails, updating databases, triggering other APIs; you name it.
But how is this any different from a usual API?
Great question! This might look very similar to a standard API at first glance, but the key difference here is the why and when these endpoints get hit. In a regular API, you're the one initiating the call, asking the server to give you something or do something for you. In the case of webhooks, it's the other way around. The server is reaching out to your endpoint to notify you about events as they happen, without you having to ask for that information. 🤯
In our Node.js example, we're setting up an endpoint that waits for incoming payloads related to specific events. Once an event happens—say, a new user signs up—the system automatically 'hooks' into our endpoint and notifies us. It's a way for apps to communicate with each other in real time, without us having to continually ask for updates.
So, to clarify: while the code may seem similar to a usual API, the use-case and workflow are what set webhooks apart. APIs are great for when you want to pull data; webhooks are the go-to when you want to be pushed updates. 🚀
Consumer App
Now that we've set up a webhook provider, let's create a simple consumer app that triggers these webhooks. We'll use Node.js and Axios for this part.
mkdir consumer
cd consumer
npm init -y
npm install axios
Create an index.js
file in the consumer app directory and start with the following:
const axios = require('axios');
// Function to trigger the webhook
async function triggerWebhook(eventType, payload) {
try {
const response = await axios.post('http://localhost:3000/webhook', {
event_type: eventType,
...payload
});
console.log(`Successfully triggered ${eventType} webhook: `, response.data);
} catch (error) {
console.log(`Error triggering ${eventType} webhook: `, error);
}
}
// Trigger different webhooks
triggerWebhook('user_signup', { user: 'JohnDoe' });
triggerWebhook('order_placed', { order: '1234' });
triggerWebhook('payment_received', { payment: '5678' });
Synchronous Calls
In a synchronous call, your application would wait for the webhook to respond before proceeding. This could make sense if your app must know the outcome of the webhook before doing anything else. However, this also makes your app more tightly coupled to the webhook server and more vulnerable to slowdowns or errors on the webhook side.
Asynchronous Calls
Asynchronous calls, on the other hand, allow your application to continue running other tasks without waiting for the webhook to respond. This is usually preferable for webhooks, as they are often "fire-and-forget" types of interactions where the result doesn't immediately impact your app. It's a more decoupled approach and generally better suited for a scalable, responsive application.
In our example
In our consumer app, I used async/await
for the Axios POST request mainly to handle the promise and catch any errors elegantly. However, we're not holding up the entire application for this webhook to respond. If you have other tasks to run, they won't be stuck waiting for this webhook.
In other words, our Axios POST request is technically asynchronous when it comes to the overall Node.js event loop. But within our triggerWebhook
function, we're waiting for the response just to handle it gracefully.
📝 Conclusion
Phew, that was quite the journey, wasn't it? We started with the basics of what a webhook is, explored how it differs from a regular API, and even built a small but meaningful example to bring it all together.
🔑 Key Points
- Webhooks are Event-Driven: Unlike APIs, where you request data, webhooks push data to you as events occur.
- Implementation: Implementing webhooks involves setting up an endpoint that listens for incoming HTTP POST requests. Your app can then react in real-time to events.
- Design Patterns: We talked about how the event-driven and observer patterns fit naturally with webhooks.
- Hands-On Example: We coded a webhook provider and a consumer app, exploring both sides of the webhook interaction.
🚀 Next Steps
So what's up next? If you're looking to dig deeper, consider exploring more advanced webhook topics, such as:
- Securing your webhooks: Make sure that the incoming requests are genuinely from the service you're integrating with.
- Retry mechanisms: If your webhook fails to trigger or times out, how should you handle it?
- Logging and Monitoring: Keep track of the incoming and outgoing webhook data for debugging and analytics.
Feel free to reach out if you have questions or if there's another topic you'd like me to cover in future posts. Until then, happy coding! 😄